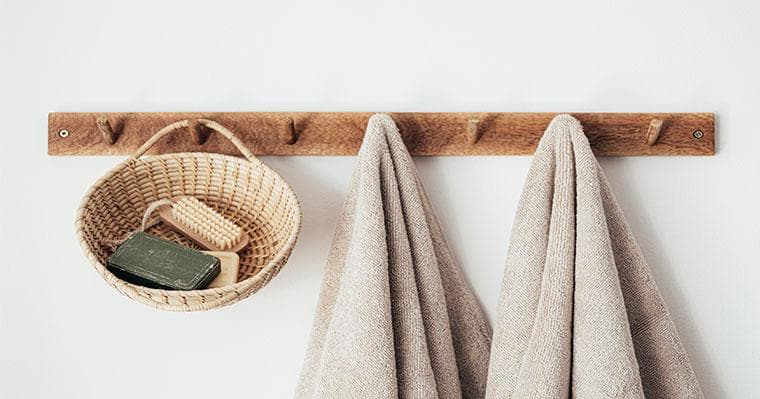
How to remove duplicates in an Javascript Array - 3 ways
Arrays are a great way to handle data, but sometimes you want to remove the duplicates from a list. You want to show a list of categories or tags being used but do not want to show every occurence. How can that be done?
1. Use Set
Using Set(), you can output an instance of unique values, essentially using this instance will delete the duplicates from the new array.
The best way to do this is create a new array based on the original array:
let pets = ['Dogs', 'Cats', 'Llamas', 'Dogs', 'Llamas'];
let uniquePets = [...new Set(pets)];
console.log(uniquePets);
Result:
['Dogs', 'Cats', 'Llamas']
2. Using the indexOf() and filter() methods
The indexOf() method returns the index of the first occurrence of the element in the array:
let pets = ['Dogs', 'Cats', 'Llamas', 'Dogs', 'Llamas'];
chars.indexOf('Dogs');
Result:
0
Therefore to use this you need to incorporate the forEach function. The duplicate element is the element whose index is different from its indexOf() value:
let pets = ['Dogs', 'Cats', 'Llamas', 'Dogs', 'Llamas'];
pets.forEach((element, index) => {
console.log(`${element} - ${index} - ${pets.indexOf(element)}`);
});
Result:
Dogs - 0 - 0
Cats - 1 - 1
Llamas - 2 - 2
Dogs - 3 - 0
Llamas - 4 - 2
To eliminate duplicates, the filter() method is used to include only the elements whose indexes match their indexOf values, since we know that the filter method returns a new array based on the operations performed on it:
let pets = ['Dogs', 'Cats', 'Llamas', 'Dogs', 'Llamas'];
let uniquePets = pets.filter((element, index) => {
return pets.indexOf(element) === index;
});
console.log(uniquePets);
Result:
['Dogs', 'Cats', 'Llamas']
But what if you want to know what elements have the duplicates? Well we just change the rule:
let pets = ['Dogs', 'Cats', 'Llamas', 'Dogs', 'Llamas'];
let uniquePets = pets.filter((element, index) => {
return pets.indexOf(element) !== index;
});
console.log(uniquePets);
Result:
['Dogs', 'Llamas']
3. Using the includes() and forEach() methods
The include() function returns true if an element is in an array or false if it is not.
The following example iterates over the elements of an array and adds to a new array only the elements that are not already there:
let pets = ['Dogs', 'Cats', 'Llamas', 'Dogs', 'Llamas'];
let uniquePets = [];
pets.forEach((element) => {
if (!uniquePets.includes(element)) {
uniquePets.push(element);
}
});
console.log(uniquePets);
Result:
['Dogs', 'Cats', 'Llamas']
As we can see there are different methods to solve a problem. Use which ever one best suits your needs.